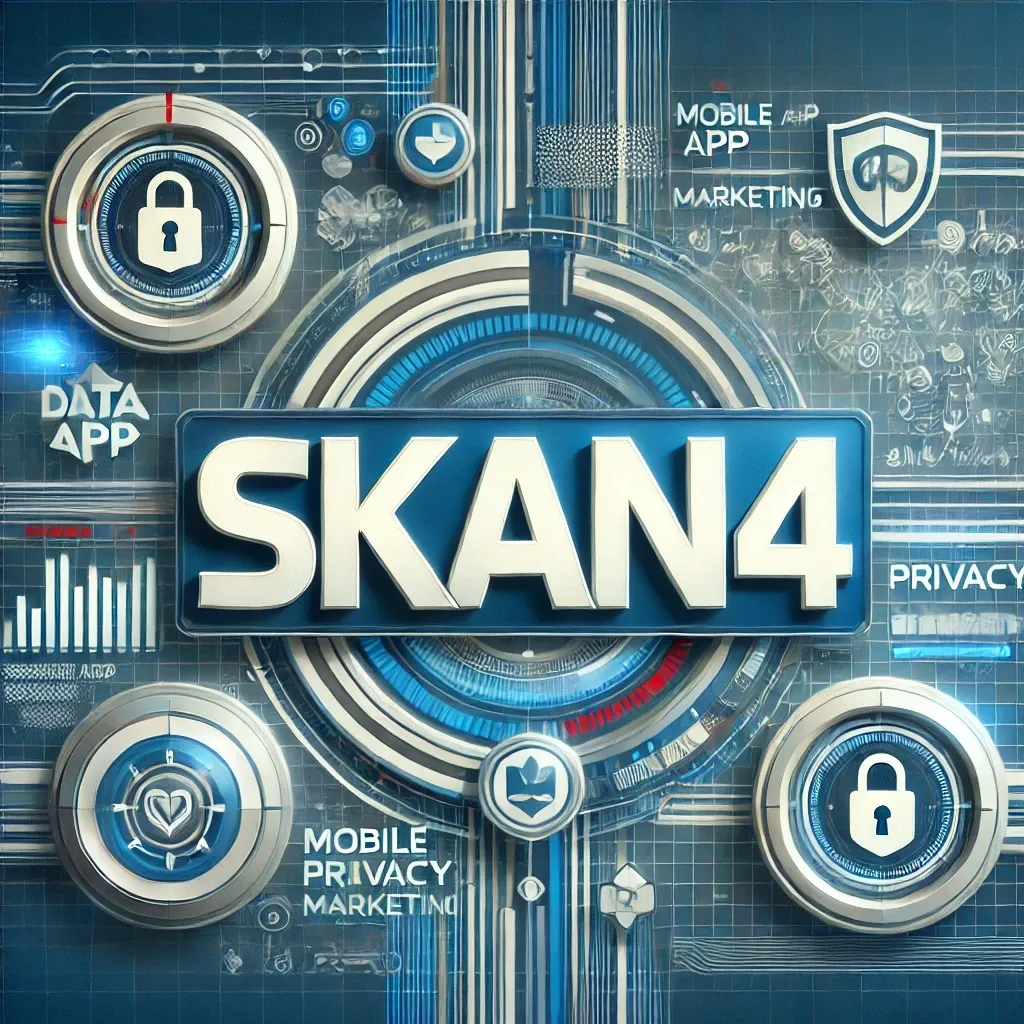
SKAdNetwork (SKAN) 4.0 isn't compulsory yet, but it's highly recommended for iOS apps and marketers. It's extremely important if you're running paid user acquisition campaigns. SKAN 4.0 provides more advanced tools to measure these campaigns' success while keeping user data safe. By using SKAN 4.0, marketers can better understand their campaign results and make smarter decisions, improving their strategies and outcomes.
Now let’s understand how SKAN 4.0 works and the implementation process.
Implementing SKAN 4.0 (for publishers)
Here is a <SWIFT> code example to illustrate the key aspects of implementing SKAN 4.0:
//Example code, not to be directly used in prod
import AppTrackingTransparency
import AdSupport
import StoreKit
class SKANManager {
static let shared = SKANManager()
private init() {}
func registerAppForAdNetworkAttribution() {
if #available(iOS 14.0, *) {
ATTrackingManager.requestTrackingAuthorization { status in
// Handle authorization status
}
}
}
func updateConversionValue(_ value: Int) {
if #available(iOS 14.0, *) {
SKAdNetwork.updateConversionValue(value)
print("Conversion value \\\\\\\\(value) updated")
}
}
// Example mapping of fine-grained events to coarse values
private func mapFineToCoarseValue(_ fineValue: Int) -> Int {
switch fineValue {
case 0...5: return 0
case 6...10: return 1
// ... other mappings
default: return 63
}
}
func trackEvent(_ eventName: String) {
if #available(iOS 14.0, *) {
let fineConversionValue = // ... your logic to determine the value (0-63)
let coarseValue = mapFineToCoarseValue(fineConversionValue)
SKAdNetwork.updateConversionValue(coarseValue)
print("SKAdNetwork conversion value updated: \\\\\\\\(coarseValue)")
}
}
}
Explanation and Usage
- <registerAppForAdNetworkAttribution()> : It's advisable to include the <AppTrackingTransparency>prompt in your application. Although not strictly part of SKAN, it sets the groundwork for user transparency.
- <updateConversionValue(_ value: Int)> : This function allows you to send your conversion data to SKAdNetwork. Keep in mind that you can update the conversion value multiple times throughout your tracking process.
- <mapFineToCoarseValue(_ fineValue: Int)> : This helper function shows how to translate detailed event tracking into more generalized values that SKAN uses.
- <trackEvent(_ eventName: String)> : Integrate this method into your existing event tracking architecture. It is critical for determining the precise conversion value to communicate.
Implementation Steps
- Integrate the Code: Add the <SKANManager> class file into your project source files.
- Call <registerAppForAdNetworkAttribution()> : Implement this call early in the app's lifecycle, such as within the <AppDelegate>.
- Implement <trackEvent()> : Make sure you call this method each time a significant in-app event, which you wish to attribute through SKAN, occurs.
- Server-Side Setup: Coordinate with your ad network partner to configure your server to appropriately receive and process postbacks from Apple.
Key Considerations
- Conversion Value Strategy: Develop and clearly define a strategy for mapping your app's events to the SKAN conversion values for accurate reporting and analysis.
- Timely Updates: Ensure that you call <updateConversionValue()> within the allowable timeframes to maintain data integrity.
- Testing is Crucial: It's essential to thoroughly test your implementation to ensure data accuracy and reliability before deploying it on a wider scale.
To stay current, search for “SKAdNetwork 4.0” or “measuring advertising effectiveness” on developer.apple.com. Regularly review new documentation and best practices as Apple releases updates.
How SKAdNetwork 4.0 Works
SKAdNetwork 4.0 brings important updates, offering more flexibility for mobile app tracking while keeping user privacy intact. Here's a simplified look at its key features:
Main features of SKAN 4.0
Privacy-focused tracking: SKAN 4.0's foundation is based on privacy. It connects app installations to advertising campaigns without disclosing user identities. The data used is anonymized and aggregated, ensuring user privacy.
Multiple conversions: A key upgrade in SKAN 4.0 is the ability to track up to three post-install activities, known as conversions. This gives advertisers a more comprehensive understanding of user behavior beyond initial installs.
Conversion values: Conversion values categorize the post-install activities you wish to track. These can range from simple classifications (low, medium, high) to more detailed categorizations, depending on the applicable privacy thresholds.
Privacy limits: Privacy limits govern the level of detail within the conversion data. Higher privacy settings offer less granular data but stronger user privacy. These limits are influenced by the number of installs an ad campaign generates.
Layered conversion values: SKAN 4.0 allows for hierarchical structuring of conversion values, enabling varying levels of detail. A general value might signify a purchase, while a more specific value could indicate the product category purchased.
Locking conversions: The ability to “lock” a conversion value prevents subsequent changes. This is particularly useful for tracking crucial events like initial purchases.
Crowdsourced data: In cases where a campaign doesn't meet the required privacy thresholds, SKAN 4.0 provides basic conversion data derived from crowdsourced information. This approach maintains the privacy of individual user data.
Attribution timelines: SKAN 4.0 retains the concept of attribution windows, which define the timeframe following an ad click during which a conversion can be attributed to that ad. Now, there are three potential postbacks, each with potentially distinct attribution windows.
How SKAN 4.0 works
Ad interaction: The process begins with a user viewing or clicking an ad from an ad network that supports SKAN.
App installs: Following the ad interaction, the user installs and opens the advertised app.
Postbacks: SKAN transmits postbacks (delayed notifications) to the ad network containing anonymized and aggregated data. Up to three postbacks are possible, each associated with a different conversion. These postbacks include the source app ID (ad network identifier), the conversion value (encoded information about app usage), and potentially a coarse conversion value (a simplified value derived from crowdsourced data if privacy limits aren't met).
Campaign optimization: Advertisers leverage this data to enhance their campaigns, concentrating on users and content that drive the desired actions.
Example
Consider a scenario where you're promoting a game app. You might define your conversions as follows:
Conversion 1 (Low) represents completing the tutorial, Conversion 2 (Medium) signifies reaching Level 5, and Conversion 3 (High) indicates making an in-app purchase. If a user installs the app, completes the tutorial, and reaches level 5 but doesn't make a purchase, you'll receive postbacks reflecting this activity, provided the privacy limits are met.
Important Notes
Delayed Data: Due to the emphasis on privacy protection, data is delayed and aggregated, precluding real-time tracking.
Limited Tracking: There is a limit of three conversions that can be tracked per install.
Less Detailed Data: Campaigns that fall short of the privacy thresholds will receive less detailed data.
Keep in mind to check Apple's official documentation for the latest details.